Go back to the contents page or the algorithms section.
Let's see how our robot navigates. Several functions are available to make navigation possible:
- move_sonar(distance)
- move_distance_forward(distance)
- make_distance_backward(distance)
- turn_right()
- turn_left()
- u_turn()
and some others to grab the ball or drop it. Most of these functions do what their name implies. Here is what some of them do:
void move_sonar(distance){
//moves to the position where the value given by the ultrasonic sensor is distance +/- 5 mm
}
void move_distance_forward(distance){
//goes straight on for distance millimeters
}
void move_distance_backward(distance){
//back-pedals for distance millimeters
}
These functions are used both in the small arena and the big arena. Let's see how they are used in the small arena. Firstly as the beginner.
void beginner_going(){}
//Starting in the beginner starting area, looking at the bottom wall.
move_sonar(930)
//goes back until the center of rotation of the robot is at half of the height of the arena
turn_left()
//turns left in order to be horizontal and looking at the closest wall
move_sonar(436)
//going backwards until the robot is close to the ball area
u_turn()
//turns around itself in order to have the claw located in the middle of the ball area
drop_ball()
//drops the ball in the ball area
//sends BALL message to the server
move_distance_backward(100)
//goes a little bit backwards
u_turn()
//turn around itself in order to watch the wall on the right of the arena
move_sonar(230)
//goes closer to the wall
turn_left()
//turns left in order to be in front of its destination area
move_sonar(230)
//goes forward until it is in the destination area
//sends NEXT message to the server
//waits for a NEXT message from the teammate
if NEXT_message_received {
beginner_return()
}
}
void beginner_return{
move_sonar(810)
//goes backward until being a little bit higher than the ball area
turn_right()
//turns right in order to be horizontal and looking at the closest wall
move_sonar(710)
//goes backward in order to arrive on the top-left corner of the ball area
scan_right()
//looks at the ball on its right and grabs it
//sends BALL message to the server
//goes horizontally again
move_sonar(230)
//moves horizontally until being closed from the right wall
turn_right()
//turns right in order to be watching at the beginner starting area (its direction)
move_sonar(230)
//goes forward until it is in the starting area
beginner_going()
//starts again
}
Here is a short representation of the trajectory of the robot as a beginner - following the code above.
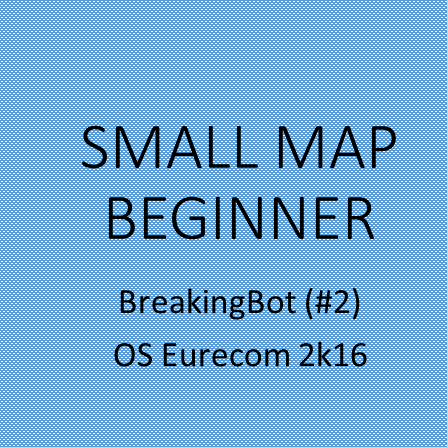
In case BreakingBot is the finisher, the pseudo-code is the one below. Note that "finisher_going" is like "beginner_return" but with a central symmetry. Likewise, "finisher_return" is like "beginner_going" with the same symmetry. :
void finisher_going(){
move_sonar(810)
//goes backward until being a little bit higher than the ball area
turn_left()
//turns left in order to be horizontal and looking at the closest wall
move_sonar(710)
//goes backward in order to arrive on the top-left corner of the ball area
scan_left()
//looks at the ball on its left and grabs it
//sends BALL message to the server
//goes horizontally again
move_sonar(230)
//moves horizontally until being closed from the right wall
turn_left()
//turns left in order to be watching at the finisher destination area (its direction)
move_sonar(230)
//goes forward until it is in the destination area
finisher_going()
//starts again
}
void finisher_return{
move_sonar(930)
//goes back until the center of rotation of the robot is at half of the height of the arena
turn_right()
//turns right in order to be horizontal and looking at the closest wall
move_sonar(436)
//going backwards until the robot is close to the ball area
u_turn()
//turns around itself in order to have the claw located in the middle of the ball area
drop_ball()
//drops the ball in the ball area
//sends BALL message to the server
move_distance_backward(100)
//goes a little bit backwards
u_turn()
//turn around itself in order to watch the wall on the right of the arena
move_sonar(230)
//goes closer to the wall
turn_right()
//turns right in order to be in front of its starting area
move_sonar(230)
//goes forward until it is in the starting area
//sends NEXT message to the server
//waits for a NEXT message from the teammate
if NEXT_message_received{
finisher_going()
}
}
Go back to the contents page or the algorithms section.
Created by Sofiène Jerbi, Thibault Petitjean (@thib774) and Florian Kohler (@FlorianKohler)
OS Course, Eurecom 2k16